Swift infinite image rotation
This snippet will be the last in our series on Swift animations for now. We will see how to set up ...
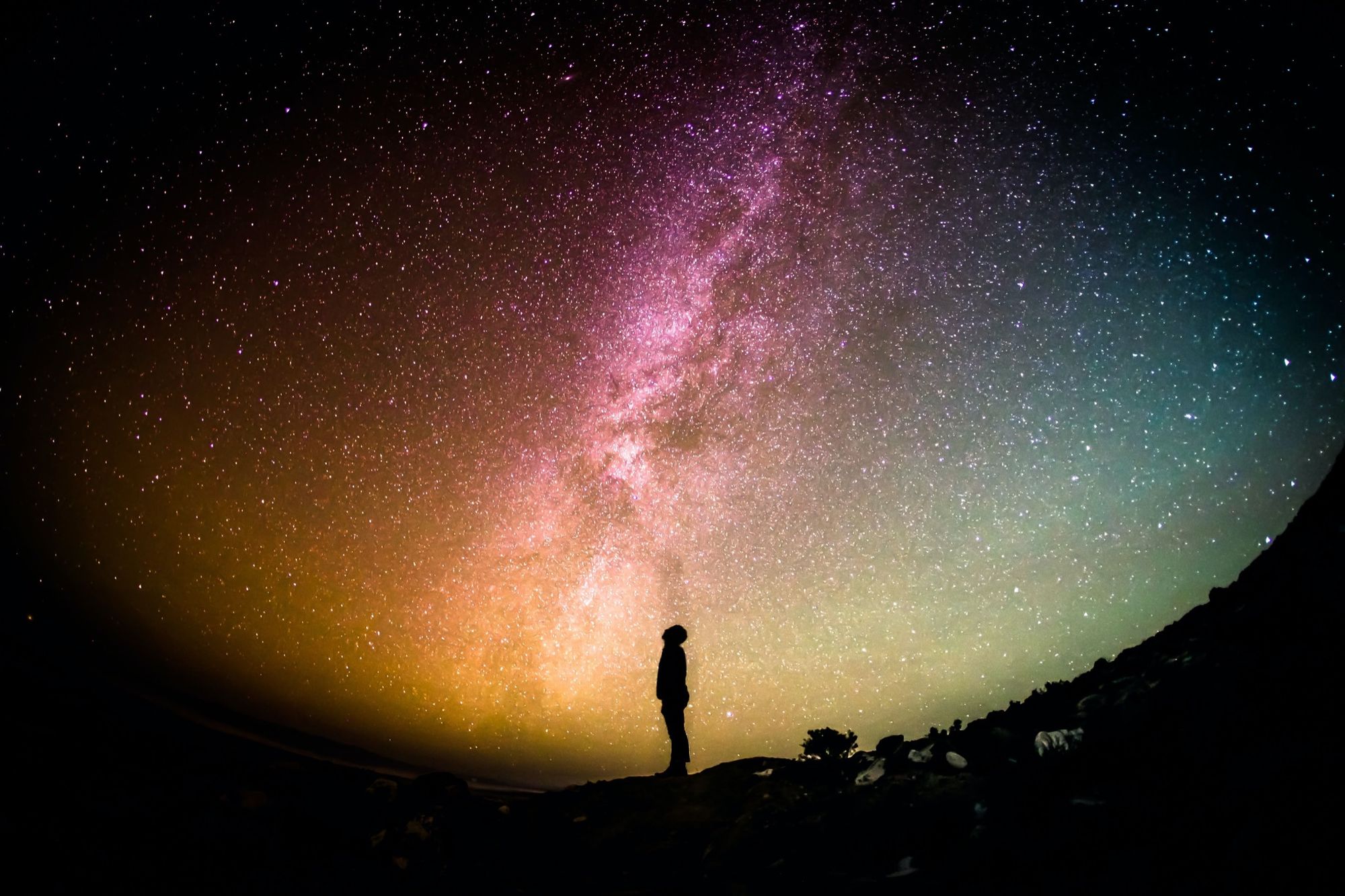
This snippet will be the last in our series on Swift animations for now. We will see how to set up the infinite image rotation. We use it for a starry background 😊.
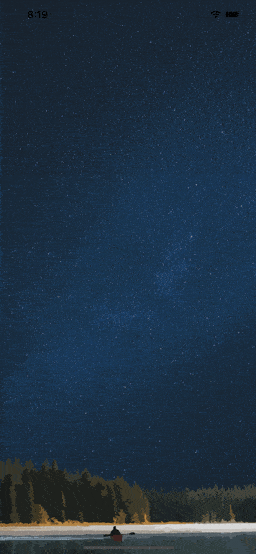
Previous articles were:
Snippet: Swift UILabel, letter animation
Here is a snippet to animate the display of a UILabel as if you were typing text on the keyboard ...
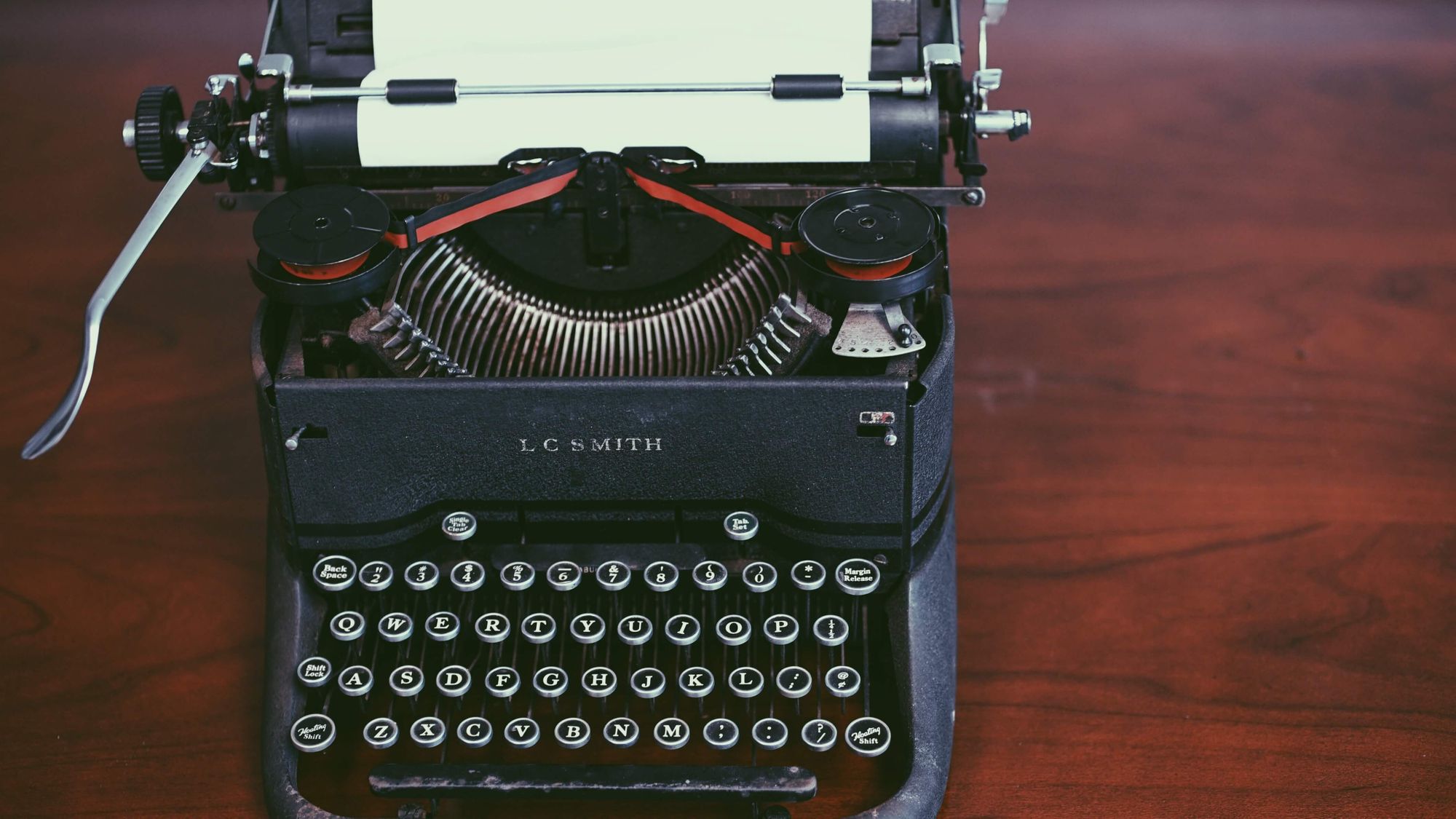
Snippet: Swift SnapKit & RxSwift animation
Here is a code snippet of a sample animation with RxSwift and Snapkit. It is not that complicated ...
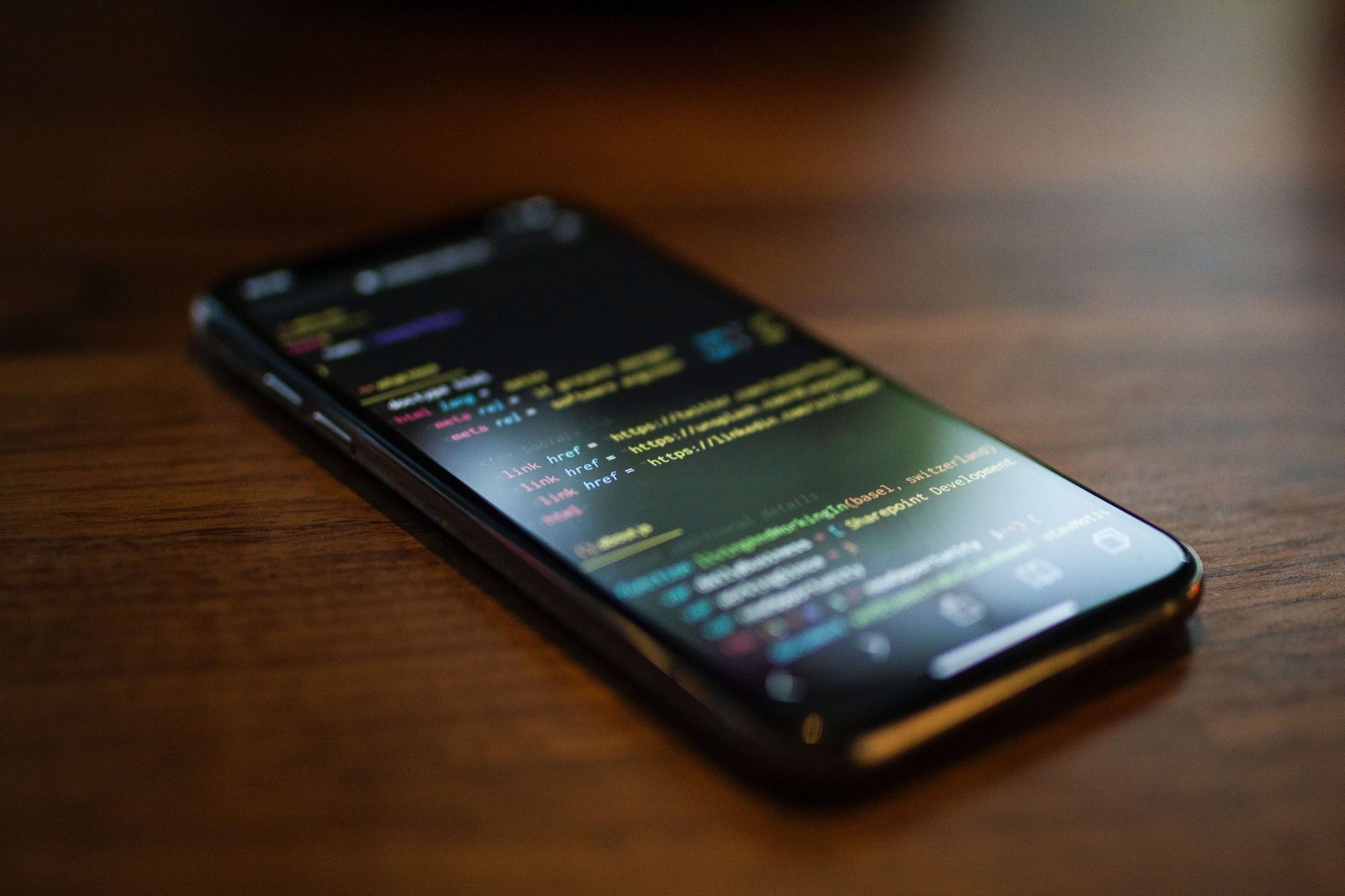
Image
let stars = UIImageView().then {
$0.contentMode = .scaleToFill
$0.alpha = 1
$0.image = UIImage(named: "stars")
$0.alpha = 0.4
}
Rotation
private func rotateImageView() {
UIView.animate(withDuration: 240, delay: 0, options: UIView.AnimationOptions.curveLinear, animations: { () -> Void in
self.stars.transform = self.stars.transform.rotated(by: .pi / 2)
}) { (finished) -> Void in
if finished {
self.rotateImageView()
}
}
}
Launch
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
rotateImageView()
}
Perfect 👏
To give you an idea, by assembling the three articles listed above, we can achieve this :